Table of content
Most asked Java Interview Questions
1. If the main method is not declared as static, what will happen?
2. What is the concept of method overloading in Java?
3. Why does Java not support multiple inheritance.
4. How can we restrict a class from being inherited?
5. Is it possible to execute Java code, even before the main method? Explain.
6. List differences between Object-oriented and object-based programming language?
7. Is it possible to overload the main method in Java?
8. What are cookies in Servlets?
9. Explain HTTP Tunneling?
10. How to write the string reversal program in Java without using the in-built function?
Basic Java Interview Questions
1. What is Java, and what makes it popular among developers?
Java is a high-level object-oriented programming language developed by Sun Microsystems. Java has some unique features, making it popular among developers. Features like platform independence, automatic memory and pointer management, object-oriented design, multithreading, and other features have made it extremely popular among developers and software companies.
2. What makes Java platform independent?
Java’s ‘Write Once, Run Anywhere’ feature allows it to be platform-independent. Once a Java program is written, it can run on any machine or operating system with Java installed.
When the code is compiled, it is compiled into an intermediate language called Bytecode. Bytecode is not dependent on any operating system or hardware. Once the code is compiled into Bytecode, it is then executed by Java Virtual Machine(JVM).
JVM is platform-dependent and needs to be installed on the platform where you want the code to run. Therefore, as long as the target platform has JVM installed, it can run any code which is written in Java, making it platform-independent.
3. Explain the differences between Java and C++.
Java |
C++ |
1. More focus on portability and simplicity |
1. It is a software layer that provides the necessary environment to run java programs. |
2. Platform independent, just needs target platform’s JVM installed |
2. It consists of the JVM, java libraries like .util and .lang, and runtime tools like java collector. |
3. Automatic Memory Management |
3. Manual memory management |
4. It is slower since code is first converted to bytecode and then converted by JVM to machine code. |
4. Faster due to direct compilation to machine code directly. |
5. Simpler Syntax |
5. Complex Syntax compared to Java |
6. Used in enterprise applications, web development, android applications etc. |
6. Used in creating system software, game development, embedded systems etc. |
4. Which features of the Java programming language makes it popular?
Java’s popularity lies in the features it offers. Here are the top features provided by Java:
- Platform Independent: Code can run on any platform
- Object Oriented: Follows the principles of OOPS
- Simple and Easy to Learn: Doesn’t include complex features such as pointers, operator overloading etc.
- Secure: Explicit access to pointers is unavailable, making it less vulnerable.
- Multi-threaded: Supports parallel computing
- Distributed Computing: In-built support for remote method invocation and protocols like HTTP and FTP.
Are you ready for your interview?
Take a quick Quiz to check it out
5. How does the JVM work?
Java Virtual Machine is a Java Runtime Environment(JRE) component. It loads, executes, and verifies the bytecode. Although it is platform-dependent, it helps make Java code platform-independent.
6. Explain the working of JIT.
JIT is a runtime component of the Java Virtual Machine (JVM) which helps convert bytecode into machine code. It is responsible for speeding up code execution which helps improve the performance and efficiency of Java applications.
It does so by recognising code which is executed frequently and converting it into machine code. This machine code is then saved in memory and whenever the repetitive block of code has to be executed again, it references it from the memory and doesn’t let JVM convert it into machine code again.
This makes the code execution efficient and fast, and saves JVM the time and resources which otherwise would have been spent in executing repetitive code.
7. Compare JDK, JRE and JVM in Java.
JDK |
JRE |
JVM |
1. It includes the full suite of tools required for developing Java applications. |
1. It is a software layer that provides the necessary environment to run java programs. |
1. JVM converts Java Code into Bytecode. |
2. Includes JRE, JVM, java compiler, java debugger and javadoc. |
2. It consists of the JVM, java libraries like .util and .lang, and runtime tools like java collector. |
2. It is platform dependent i.e it has different version of Mac, Linux and Windows. |
8. Explain the following syntax: public static void main(String args[]) in java?
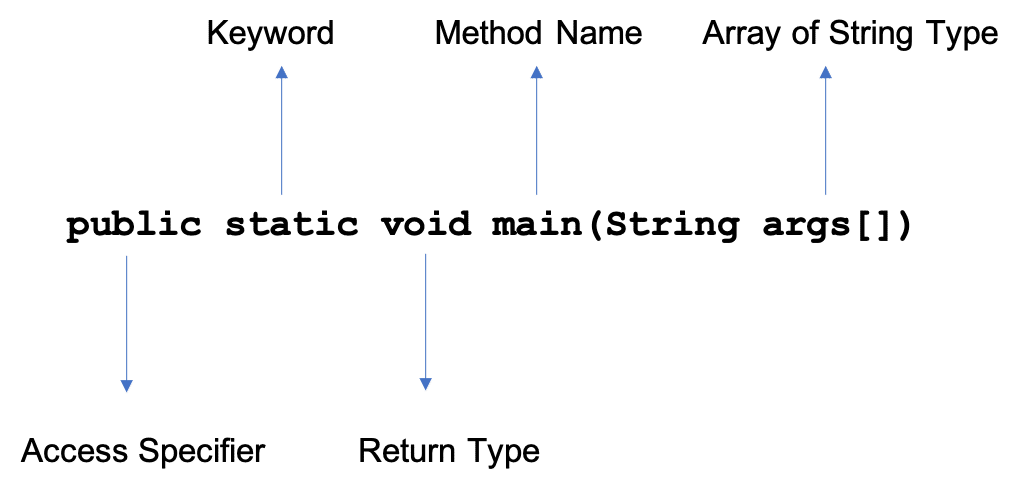
Every Java program needs a main method. When you compile a Java program, the JVM looks for the main method with the exact same syntax. Let’s break down the syntax to understand it better.
Public: The access specifier ‘public’ helps JVM access the method from outside the class.
Static: The static keyword helps to make a method or variable belong to a class, and not to an instance of a class i.e it’s object. This is useful because even if an object is instantiated from the class, the main method will remain global and not instantiate again, hence not confusing the JVM.
Void: This is a return type, and means the main method is not returning any value to the caller. In this case, it’s the JVM.
main(String args[]): This is the main method, with parameters of args[]. args[] helps to catch any command line arguments passed while launching the program.
9. If the main method is not declared as static, what will happen?
The program will compile, but you will get the following runtime error.
Error: Main method is not static in class Example, please define the main method as: public static void main(String[] args)
10. Which memory areas are allocated by JVM?
JVM is the core part of the Java Platform, and it is responsible for running Java programs. To execute the programs, JVM requires to store the instructions, variables etc of the program in the memory. JVM’s memory is divided into specialised memory areas, let’s understand them:
- Method Area (Metaspace): This area of the memory saves the class metadata, method metadata, field metadata and static variable. After Java 8 implementations, this area is called metaspace.
- Heap: This is the largest memory area, and it stores objects and their associated instance variables.
- Java Stacks(Thread stacks): It is a runtime memory area which is created for each thread. It stores local variables, method arguments etc. It is designed to handle method execution and local variables.
- PC Registers: The program counter register help point to the current thread being executed. After executing an instruction the PC points to the next instruction.
- Native Method Stacks: It is a memory area in JVM which is reserved for native methods written in languages other than Java. Like C/C++. It helps java interact with system level APIs which are not written in Java.
11. What are the different kinds of data types in Java?
Java primarily has two kind of data types:
- Primitive Data Types: They are the most basic data types, they have simple values and are not objects. Example: byte, short, int etc.
- Non-Primitive Data types: These are also called reference types and are used to store references to objects references to objects in memory. Example: Strings, Arrays, Classes etc.
Build Your Reputation as a Java Expert
Join the Best Java Training Program
12. What are Primitive and Non-Primitive data types in Java?
Primitive data types: Primitive data types are the most basic data types in java. Examples of primitive data types include: short, int, boolean, char etc. The size of these data types are fixed, and they are stored in stack data structures for faster access. Their value cannot be null, the default value for integer is 0 and for booleans is false.
Objects or Non Primitive Data Types: Non Primitive data types or objects are instances of classes representing complex or user defined data. It is stored in the heap memory. Examples of objects data type include: array, string, list etc. The size of this data type is not fixed, and depends on the object’s structure and fields. Their default value is null.
13. Why can’t we use pointers in Java?
Pointers allow direct access to memory, and is a key feature of C/C++, however Java’s design intentionally disallows direct access to memory and therefore it does not make use of pointers. This design decision is to align Java to be a safe, simple, platform independent and secure programming language.
But how, does use of pointers be a bad thing? Let’s understand:
- Safe: Direct access to memory via pointers can lead to a lot of vulnerabilities such as unauthorized memory access, memory corruption, overflows etc. Java automatically manages the memory, hence no room for human error.
- Simple: Since java has automatic memory management, it is more simple to use.
- Platform Independent: Different systems have different memory layouts, Java abstracts the memory management, and manages it via JVM. Therefore the same code, can run on any platform.
- Secure: Restricted memory access and automatic memory management promotes better security.
14. What are Classes and Objects in Java?
A class is the blueprint of an object and object is derived from a class with all its properties.
Let’s take a real world example to understand this:
There are so many models of cars, for example Mercedes, BMW, Audi etc. These models can be considered as objects. They have been derived from a blueprint which is a “car”.
Let’s understand car as a class or blueprint.
A car has 4 wheels, it has an engine, it has brakes, wipers, headlights etc. These features of a car are called the properties of a car.
An object of this class ‘car’, will have all these properties. Cars like BMW, Mercedes and Audi, all have these properties. Hence, we call them objects of the class car.
Similarly in Java, we can define a class with custom functions, variables, constructors etc. And whenever, we define an object which is derived from a class, it inherits all it’s properties such as variables, methods etc.
Here’s an Example code to better understand this.
Here, obj is derived from class Sample. The moment obj is initialized, it will print “Hello from Sample Class!!.” This is because it derives the properties of the sample class.
15. Explain the concept of Objects in java.
An object is a blueprint of a class. It is a real world entity which holds the data and behavior inherited from its class. Syntax for creating an object: Sample obj = new Sample(); Here sample is the name of the class, obj is the object. New keyword has to be used whenever creating an object, and Sample() is the constructor of the class.
Get 100% Hike!
Master Most in Demand Skills Now!
16. Why are wrapper classes needed in Java?
We need wrapper classes to convert primitive data types to objects. They are required for special use cases such as follows:
- Working with Collections: Collections in java work with objects and not primitive data types, so wrapper classes help convert primitive data types to collections.
- Converting Data Types: Wrapper classes can help convert Strings to primitive data types.
- Utility Methods: Wrapper classes have useful utility methods, which if we want to use, then we have to convert primitive data type to object.
- Autoboxing and Unboxing: Whenever java wants it can automatically convert between primitive data types and their wrapper objects.
17. Explain the concept of Class variables.
Class variables are declared inside the class with the keyword ‘static’. They are shared among all the objects of a class. A non-static variable gets initialised with every instantiation of an object. However static variables are not tied to any object. So any modification done to them, is prevalent for all the objects derived from that class.
Let’s take an example to understand this.
Here, as you can see from the output. Static variables are common between the two objects a and b. Both of them perform an increment operation on it, and the value gets incremented to 12. Alternatively, the non-static variables are not common between objects, each object has its own copy which also reflects in the output. We incremented the variable once from each variable, but because each object had its copy, the value got incremented by only 1.
18. Explain the concept of Inheritance in Java.
Inheritance helps a child class acquire the properties and behaviour of its parent class. It is similar to how kids get a lot of traits from their parents, for example their looks, behaviour etc.
Another real world example would be Vehicles. All vehicles inherently have the same properties such as tyres, engine etc. But each vehicle type has its own unique features.
Similarly in java, when you create a class, and want to inherit the properties of it in another class, we make use of inheritance.
Take a look at the code below:
In the code, there is a parent class Vehicles. It has three functions, tyres(), engine() and doors(). We create a NewCar class which inherits the Vehicles class using the ‘extends’ keyword.
Now, NewCar will have all the properties of the Vehicle class which includes its methods.
In the main method, we created an object of the NewCar method, following is the output.
19. Explain the following variables in Java:
a. Instance Variables
b. Local Variables
c. Class Variables
Instance variables are declared inside the class but outside the methods. So basically these are accessible by all the methods of that class. The default value for numeric types is 0, for boolean false and null for object references.
Example:
Local variables’s scope is limited to the function, constructor, or block of code, so no other function can access those variables. These variables don’t have any default value.
Example:
Class variables are also known as static variables in Java, defined in a class using static keywords. These variables are stored in static memory and can be called in a program using the class name. The default values are the same as the instance variables.
Example:
The main difference between Instance variables and Class Variables is that the instance variables belong to only a specified class in which they are declared. While the class variables belong to classes and subclasses.
20. Is Java a pure object-oriented programming language?
No, Java is not a pure object-oriented programming language because it uses primitive data types like char, boolean, int, short, long, double etc. and also supports the concepts of static methods and variables, which can be directly accessed with class name. A pure object oriented programming language treats everything as an object, however java uses both Object oriented programming and procedural programming language’s features.
21. List the default values of byte, float, double, and char data type in Java.
Here are the default value of the data types:
- Byte: 0
- Float: 0.0f
- Double: 0.0d
- Char: ‘\u0000’
Are you ready for your interview?
Take a quick Quiz to check it out
Java Interview Questions for Freshers
22. What are the differences between StringBuffer and StringBuilder in Java programming.
StringBuffer |
StringBuilder |
StringBuffer methods are synchronized. |
StringBuilder is non-synchronized. |
StringBuffer is thread-safe. |
StringBuilder is not thread-safe. |
The performance is very slow. |
The performance is very fast. |
23. What is the concept of method overloading in Java?
When a Java program contains more than one method with the same name but with different argument types, it is called method overloading.
Java resolves this problem by checking the argument types or count,, basis the type of argument and count of argument, it calls the respective method.
For Example
Here when we call the function based on the argument passed, the respective method will be called, so if we pass 10, it will call the method that accepts integer as an argument, and if we pass a string, it will call the method that acceptsstring as an argument.
Watch this Java Interview Questions video:
24. List the differences between an ArrayList and a Vector.
ArrayList |
Vector |
An ArrayList is not synchronized. |
A vector is synchronized. |
An ArrayList is fast. |
A vector is slow as it is thread-safe. |
If an element is inserted into an ArrayList, it increases its array size by 50 percent. |
A vector defaults to doubling the size of its array. |
An ArrayList does not define the increment size. |
A vector defines the increment size. |
An ArrayList uses an Iterator for traversing. |
Except for the hashtable, a vector is the only other class using Enumeration and Iterator. |
25. Explain Iterator vs Enumeration.
Iterator |
Enumeration |
Iterator is an interface found in the java.util package. |
Enumeration is a special data type that allows you to define a fixed set of constants. |
Uses three methods to interface:
- hasNext()
- next()
- remove()
|
Uses two methods:
- hasMoreElements()
- nextElement()
|
Iterator method names have been improved. |
The traversing of elements can only be done once per creation. |
Become an Java Expert with Our Free Course
Achieve Your Goals with this Free Training
26. What are the differences between the inner class and the subclass.
Inner Class |
Subclass |
An inner class is a class that is defined within another class. |
A subclass is a class that inherits properties from another class called the superclass. |
It provides access rights for the class, which is nesting it, which can access all variables and methods defined in the outer class. |
It provides access to all public and protected methods and fields of its superclass. |
27. Is it possible to execute Java code, even before the main method? Explain.
Yes, we can execute code even before the main method. It can be done using two ways:
- Static Initialisers
- Static Variable Initialisation
Let’s understand static initialisers first. We can create a static block of code, with the code which we want to execute before the main method. Any statements within this static block of code will get executed before the main method.
Example
This happens because when the JVM loads the class, it executes the static block of code first by design, even before the main method. One can have multiple static blocks, they will execute in the sequence you define them in.
Next, let’s understand Static Variable Initialisation: Static variables can initialise another static method, thus making the code run before the main method, let’s understand using an example.
Here, the method intellipaat is initialised by the static variable x.
28. How can we restrict a class from being inherited?
There are various ways through which we can restrict inheritance for a class, listed them below
- By using the final keyword
- By using private constructors
- By using sealed class
- By using final keyword: if you mark a class as final, it cannot be extended, below is the code for the same.
Here, you will get an error. Since the ‘Base’ class is ‘final’, and hence it cannot be inherited. Here, if you remove the ‘final’ keyword from the ‘Base’ class, the code will work as intended.
- By using private constructors: if we make the constructor private of the class, then it cannot be inherited by another class. This way it cannot be instantiated outside the class.
Example
Here, we will get an error since the display method in the Base class is private, hence it cannot be instantiated. If we remove the keyword ‘private’ from the method, the code will work as expected.
- By sealing the class: By using the seal keyword, we can restrict which classes can inherit the base class. Let’s understand it using an example:
Here the class which wants to inherit properties from a sealed class, needs to have one of the following access specifers: final, non-sealed or sealed
29. Why does Java not support multiple inheritance.
Java doesn’t support multiple inheritance because of two reasons:
- Ambiguity:
- Simplicity
Example:
Intellipaat1 and Intellipaat2 test() methods are inheriting to class C. So, which test() method class C will take?
As Intellipaat1 and Intellipaat2 class test () methods are different, here we would face ambiguity.
30. Can a constructor be inherited? Is it possible for a subclass to call the parent’s class constructor?
We cannot inherit a constructor in Java, However, a subclass can call the parent’s constructor using the super keyword. We do this to initialise inherited fields in the parents class by creating an instance of a subclass. By calling the parent constructor, we ensure that the parent class is initialised correctly.
31. Explain a ClassLoader in Java.
It is a part of the Java Runtime Environment which is used to dynamically load the class files into the Java Virtual Machine(JVM). The classloader triggers whenever a Java file is executed.
There are three types of classloaders in Java:
- Bootstrap Classloader: The bootstrap loader is the superclass of extension classloader, which loads the standard JDK files from rt.jar and other core classes. The rt.jar file contains all the package classes of java.net, java.lang, java.util, java.io, java.sql, etc.
- Extension Classloader: The extension classloader is known as the parent of the System classloaders and the child of the Bootstrap classloader. It only loads the files available in the extension classpath, which includes ‘dirs/JRE/lib/ext directory. If the file is unavailable in the mentioned directory, the extension classloader will delegate it to the System classloader.
- System/Application Classloader: It loads the classes of application type available in the environment variable such as -classpath, or -cp command-line option. The application classloader generates a ClassNotFoundException if the class is unavailable in the application classpath.
32. Explain the working of == operator in Java?
The == operator in Java is used to compare primitive, object references and a lot more. It’s behaviour differs from case to case basis. Let’s understand from the table below:
Context |
What == compares? |
Primitives |
Values |
Object References |
Memory Locations |
String Literals |
Memory Locations in string pools |
Wrapper Objects |
Memory locations (Cached or not) |
33. Explain the try-catch block in Java?
A try-catch block in Java is used to implement exceptional handling. The code that may throw exceptions has to be written inside the try block, and if any exception occurs, catch it and work on it in the corresponding catch block.
34. Differentiate between checked and unchecked exceptions in Java.
The compiler checks checked exceptions at compile time, meaning that programmers have to handle such exceptions with try-catch blocks or declare them using the method signature. Unlike checked exceptions, unchecked exceptions are not checked at compile-time, hence they do not require explicit handling by a programmer.
35. Explain the meaning of ‘final’ keyword in Java? Give example
Java uses the final keyword to declare constants, prevent method overriding, and create immutable classes. For example, final int MAX_SIZE = 100; declares a constant MAX_SIZE whose value is 100.
36. Can you do multithreading in Java?
Yes, multithreading is part of Java. It provides built-in support for creating and managing threads, thereby enabling multiple tasks to be executed concurrently within one program.
37. Explain the use of ‘super’ keyword in Java?
Super is used in the Java programming language to refer to the superclass of an object being manipulated. It can also be used as a bridge between superclass methods/constructors and subclass methods/constructors. For instance, super.methodName() invokes the method defined by the superclass.
38. What are abstract classes in Java? Give an example.
Java Abstraction refers to encapsulating only the function ability and behavior of methods while not exposing their implementation details to the user. The object design used here focuses on what an object does rather than on how it performs that function. For instance, the List interface in Java is an example of abstraction because it describes general operations for lists without defining how they are implemented by specific list classes such as LinkedList and ArrayList.
39. Can you save a .java file without a name?
Yes, we can save a java file with an empty .java file name. You can compile it in the command prompt by ‘javac .java’ and run it by typing ‘java classname’. Here is an example of such a program:
Once the code has been saved, you need to launch the command prompt and navigate to the file’s directory. After that, you need to write “javac .java” to compile the program and “java A” to execute it.
40. List differences between Object-oriented and object-based programming language?
Following are the key differences between the object-oriented and object-based programming languages:
Object-oriented programming language |
Object-based programming language |
Object-oriented programming languages support all the features of OOPS, including polymorphism and inheritance |
Object-based programming language does not support inheritance or polymorphism |
Doesn’t support built-in objects |
Support built-in objects like JavaScript has the window object |
Example: C#, C++, Java, Python, etc. |
JavaScript and Visual Basic are examples of object-based programming language |
41. Is it possible to declare static variables and methods in an abstract class?
Yes, we can declare static variables and methods in an abstract class by inheriting the abstract class. In java, the static variables and methods can be accessed without creating an object. You can simply extend the abstract class and use the static context as mentioned below:
42. Explain Aggregation in Java?
Aggregation in Java represents a relationship where one class contains the reference of another class. In other words, it refers to a one-way relationship between two objects where the aggregate class has the instance of another class it owns. The aim is to have classes which are loosely coupled with each other.
For example, in the program below the aggregate class student have a one-way relationship with the class Address. Here each student having an address makes sense, but address having the student doesn’t make any sense. So, instead of inheritance, the aggregation is based on the usage of the classes.
The output of the above program will be:
Student details:
Name: Sam
Reg_no: 111
Address: Paper Mill Road, SRE, UP, India
Student details:
Name: Alex
Reg_no: 112
Address: Service Road, BNG, KA, India
43. Explain the concept of composition in Java?
Composition refers to a relationship between two classes/objects, where the two objects are tightly coupled and can’t exist without the existence of each other, this concept is known as composition.
In simple words, the composition is a kind of aggregation used to describe the reference between two classes using the reference variable.
For example, a class student contains another class named address. So, if the class address cannot exist without the class student, then there exists a composition between them.
44. Is it possible to overload the main method in Java?
Yes, you can overload the main() method in Java using the concept of method overloading.
Below is an example of main() method overloading:
This happens because the JVM only recognise the main method with (String args[]). If you need to call other overloaded main methods you have to call them explicitly.
Here is the code if you want to do so,
45. List the differences between constructor and a destructor?
Below is the difference between a constructor and a destructor based on various parameters:
Constructor |
Destructor |
A constructor is used to allocate the memory. |
A destructor is used to deallocate or free up the memory. |
May or may not accept arguments. |
Destructors don’t accept any arguments. |
class_name(arguments){
} |
~class_name(){
}; |
The constructor is called as soon as the object is created. |
Called at the time of program termination or when the block is exited. |
Constructors are executed in successive order, meaning from parent to child class. |
Destructors are called in the reverse order, meaning from child to parent class. |
Can be overloaded. |
Cannot be overloaded. |
46. Can you define static methods inside a java interface?
Yes, a Java interface can have static methods, but we cannot override them as they’re static. So, we have to define the static methods in the interface and only perform the calling party in the class.
Below is an example of a static method in Java interface:
Output :
error: can't find main(String[]) method in class: A
The above interface has a static method and a default method that can be executed without affecting the class that implements it. However, there is no use in creating the static methods in the Java interface as we cannot override the function by redefining it in the main() method.
47. What is a JAR file in java?
Java Archive(JAR) is a file format that aggregates many files into a single file similar in a format similar to a ZIP file. In other words, the JAR is a zipped file comprised of java lass files, metadata, and resources like text, images, audio files, directories, .class files, and more.
Java Interview Questions for Experienced Developers (3 – 5 Years)
48. Explain the differences between method overriding and method overloading.
Method overloading is using a single class that has two or more methods with the same name but different parameters. It is solved at compile-time based on the number and types of arguments given. One more instance exists when a subclass provides the specific implementation for some method already defined in its superclass, this is called method overriding, which is used to achieve runtime polymorphism.
For example
49. What is data encapsulation in java?
Encapsulation in Java is the act of keeping together data and methods that are part of a class to hide the internal state from direct access. The reason behind this approach is to ensure data integrity and promote abstraction by exposing only essential details of the class’s behavior through public methods.
To achieve data hiding, encapsulation restricts direct access to an object’s internal state, thus ensuring data integrity. By doing this, it makes abstraction possible as it only exposes some critical behaviors about the object via public methods, thereby enabling simpler interaction and decreased complexity.
50. Explain the keyword ‘final’ in java.
The final keyword can be added before a variable declaration, any method header, or preceding the class keyword so as not to allow changing their value, to prevent them from being overridden, or before words like “extends” in order not to let inheritance happen.
51. List the differences between ArrayList and LinkedList in Java?
ArrayList is a dynamic array that stores elements and supports fast random access. It is better suited for element traversal and frequent access. Conversely, LinkedList is a linked list that stores its elements in the form of nodes, making it very suitable for high-speed insertion and deletion operations. Therefore, it can be useful where there are many additions or removals.
If you want fast access and traversal through elements, then use an ArrayList; otherwise, for fast insertion or deletion of elements, use LinkedList.
52. What is a constructor in Java? Explain the different types.
There are two types of constructors in the Java programming language:
- Parameterized Constructor: A constructor that accepts arguments or parameters is known as the parameterized constructor. It initializes the instance variables will the values passed while creating an object.
Below is an example of the parameterized constructor:
The output of the above program would be:
Constructor called
Class A function called
The value of instance variables are:
Name: null
Roll No: 0
- Non-parameterized/Default constructor: The constructors with no parameters or arguments are known and non-parameterized constructors. In case you don’t define a constructor of any type, the compiler itself creates a default constructor which will be called when the object is created.
Below is an example of a non-parameterized constructor:
53. What is a Default constructor used for in Java?
If no constructor is available in the class, the Java compiler creates a default constructor and assigns the default value to the instance variables.
For example:
54. Explain the use of copy constructor in Java.
Java does not support a copy constructor. However, we can manually create one. You can copy the values of one instance to another by copying the instance variables of one object to another. Below is an example of such a method:
55. List the differences between aggregation and composition.
Below are the key differences between aggregation and composition in Java:
Aggregation |
Composition |
The parent class has a one-way relationship with the child class. |
In composition, the parent class owns the child class |
Aggregation is denoted by an empty diamond in UML (Unified Modeling Language) notation |
Denoted by a filled diamond in the UML notation |
Child class has its own life cycle |
Child class doesn’t have a lifetime |
Aggregation is a weak relationship. For example, a bike with an indicator is aggregation |
Composition is a stronger relationship. For example, a bike with an engine is a composition |
56. Explain the purpose of object cloning in Java.
Object cloning means creating the exact copy of an existing object. In java programming, object cloning can be done using the clone() method. To create the object clone, you should implement the java.lang.Cloneable interface, otherwise the clone() method generates a CloneNotSupportException.
The syntax for the clone method is:
protected Object clone() throws CloneNotSupportedException
57. Explain various exception handling keywords in Java.
Java language has three exception handling keywords:
- try: whenever a code segment has a chance of an error or any abnormality, it can be placed inside the try block. In case of any error, the try block will send the exception to the catch block.
- catch: catch handles the exception generated in the try block. It comes after the try block.
- final: The final keyword ensures that the segments execute despite the errors and exceptions processed by the try & catch block. The final keyword comes either after the try block or both the try and the catch block.
58. List the differences between this() and super() keyword in Java.
this() |
super() |
Represents the present instance of a class |
Represents the current instance of the parent class |
Calls the default constructor |
Calls the base class constructor |
Used to point to the current class instance |
Used to point to the instance of the superclass |
59. Is it possible to import the same package/class twice? Will the JVM load the package twice at runtime?
A package or class can be inherited multiple times in a program code. JVM and compiler will not create any issue. Moreover, JVM automatically loads the class internally once, regardless of times it is called in the program.
60. What are annotations in Java?.
- Java annotation is a tag that symbolizes the metadata associated with class, interface, methods, fields, etc.
- Annotations do not directly influence operations.
- The additional information carried by annotations is utilized by Java compiler and JVM.
61. List the differences between HashMap and HashTable in Java?
These two are used to save key/value pairs, but with some differences:
- HashMap does not have a synchronization feature, making it not thread-safe, while HashTable does.
- HashMap allows null keys as well as values, but HashTable does not allow any null key or value.
Generally, HashMap is preferred in non-thread-safe scenarios where performance is important; on the other hand, HashTable is recommended for multi-threaded environments where thread safety is required.
62. Explain the use of access modifiers in Java.
Public, protected, default (no modifier), and private are four access modifiers in Java.
- Public: access from anywhere
- Protected: access to the same package or other packages (subclasses)
- Default (no modifier): Only access in the created package.
- Private: No one else but the declaring class will be able to obtain it.
Use public if you want your member to be accessed from anywhere, protected when you wish to restrict access to subclasses and classes within the same package, default when you want access at the package level, and private when you only want access within the declaring class.
63. Explain memory management and garbage collection in Java.
Garbage collection is Java’s automatic memory management system. It runs as a process that recovers memory from objects that will never be used again. Garbage collection is done by JVM, it does tasks like allocating memory for objects, garbage collecting unused objects, and optimizing memory usage for performance enhancement. Various types of memory areas are used by JVM such as heap, stack method area, and native method stacks to help keep its memory efficient.
64. List the differences between static loading and dynamic class loading.
- Static loading: In static loading, classes are loaded statically with the operator ‘new.’
- Dynamic class loading: It is a technique for programmatically invoking the functions of a class loader at runtime. The syntax for this is as follows:
Class.forName (Test className);
65. Explain the difference between Struts 1 classes and Struts 2 classes.
Struts 1 actions are singleton. So, all threads operate on the single action object and hence make it thread-unsafe.
Struts 2 actions are not singleton, and a new action object copy is created each time a new action request is made and hence it is thread-safe.
66. What is JAXP and JAXB.
JAXP: It stands for Java API for XML Processing. This provides a common interface for creating and using DOM, SAX, and XSLT APIs in Java regardless of which vendor’s implementation is actually being used.
JAXB: It stands for Java API for XML Binding. This standard defines a system for a script out of Java objects as XML and for creating Java objects from XML structures.
67. What is enumeration?
It is a special data type, which is used to define a fixed set of constants to a variable. It is usually used, when the data that one is dealing with is not dynamic in nature.
68. What methods are available in Java to find the actual size of an object on the heap?
In the Java programming language, you cannot accurately determine the exact size of an object located in the heap.
69. What’s the base class of all exception classes?
Java.Lang.throwable: It is the superclass of all exception classes, and all exception classes are derived from this base class.
70. What is the use of a vector class?
A vector class provides the ability to execute a growable array of objects. A vector proves to be very useful if you don’t know the size of the array in advance or if we need one that can change the size over the lifetime of a program.
71. Explain the difference between transient and volatile keywords in Java.
Transient: In Java, it is used to specify whether a variable is not being serialized. Serialization is a process of saving an object’s state in Java. When we want to persist the object’s state by default, all instance variables in the object are stored. In some cases, we want to avoid persisting a few variables because we don’t have the necessity to transfer across the network. So, we declare those variables as transient.
If the variable is confirmed as transient, then it will not be persisted. The transient keyword is used with the instance variable that will not participate in the serialization process. We cannot use static with a transient variable as they are part of the instance variable.
Volatile: The volatile keyword is used with only one variable in Java, and it guarantees that the value of the volatile variable will always be read from the main memory and not from the thread’s local cache; it can be static.
72. Can Map interface extend the Collection interface in the Java Collections Framework?
No, The Map interface is not compatible with the Collection interface, because Map requires a key as well as a value, for example, if we want to add a key–value pair, we will use put(Object key, Object value).
There are two parameters required to add an element to HashMap object. In Collection interface, add(Object o) has only one parameter.
The other reasons are: Map supports valueSet, keySet, and other suitable methods that have just different views from the Collection interface.
73. What is the use of synchronized block.
We use the synchronized block because:
- It helps lock an object for every shared resource.
- The scope of the synchronised block is smaller than the method.
74. What is the difference between Stack and Heap Memory in Java?
The key differences between Heap and Stack memory in Java are:
Stack Memory |
Heap Memory |
Smaller in size. |
Larger in size. |
Exists until the end of the thread. |
Lives from the start till the end of application execution. |
Objects stored in Stack can’t be accessed by other threads. |
Globally accessible. |
Follows LIFO(Last In First Out) order. |
Dynamic memory allocation. |
The variables in Stack memory are only visible to the owner thread. |
Heap memory is visible to all the threads. |
Continuous memory allocation. |
Random order memory allocation. |
Less flexible, one cannot alter the allocation memory. |
Users can alter the allocated memory. |
Fast access, allocation, and deallocation. |
Slow access, allocation, and deallocation. |
75. What is an interface in Java?
Java interface is a completely abstract class referred to as a collection of abstract methods, static methods, and constants. It acts as a blueprint of a class that groups related methods or functions with empty bodies.
Below is an example of a java interface:
76. Explain the difference between abstract class and interfaces?
Below are the major differences between the abstract class and an interface:
Abstract Class |
Interface |
Can provide complete, default, or only the details in method overriding |
Doesn’t provide any code, the user can only have the signature of the interface. |
Have instance variables. |
No instance variables. |
An abstract class can be public, private, or protected. |
The interface must be public. |
An abstract class can have constructors. |
No constructors are available in the interface. |
A class can inherit only a single abstract class. |
A class can implement one or more interfaces. |
Abstract classes are fast. |
Interfaces are slower as they have to locate the corresponding methods in the class. |
77. What is the purpose of servlets in java?
It’s a server-side technology used to create web servers and application servers. We can understand Servlets as the java programs that handle the request from the web servers, process the request, and reverts to the web servers after the response is generated.
Several packages like javax.servlet and javax.servlet. HTTP provides the appropriate interfaces and classes that help the user to create their servlet. All the servlets should implement the javax.servlet.Servlet interface, plus other interfaces and classes like the HttpServlet, Java Servlet API, and more.
The execution of a servlet involves six distinct steps:
- The clients initiate the request by sending it to the web server or application server.
- The web server receives the request from the clients.
- The servlet processes the request, carrying out the necessary computations, and generates the corresponding output.
- Subsequently, the servlet sends the processed request back to the web server.
- Finally, the web server dispatches the request to the clients, and the resulting output is displayed on the client’s screen or any other designated device.
78. Explain the use of a Request Dispatcher in Java?
The request dispatcher serves as an interface utilized to effectively redirect requests to various resources within the application itself. These resources may include Java Server Pages (JSP), images, HTML files, or other servlets. Moreover, it facilitates the integration of responses from one servlet into another, guaranteeing that the client receives output from both servlets. Additionally, it allows for the seamless forwarding of client requests to the next servlet in the specified sequence.
There are two methods defined in a Requestdispatcher interface:
- void include(): It includes the content of the resource before sending the response.
- void forward(): the method forwards the request from one servlet to another resource like the JSP, image, etc on the server.
79. What are cookies in Servlets?
Cookies are text files which are stored on the client side (web browser) and maintain information about the state or session information. They are required by servers, since HTTP protocols are stateless in nature. They help the server to know the user preferences, session information etc.
Cookies have a size limitation of 4KB. They can be cleared or disabled by the user.
80. List the differences between ServletContext vs. ServletConfig?
The difference between ServletContext and ServletConfig is as follows:
ServletContext |
ServletConfig |
It is a global object, which helps get information/configuration for the entire application. |
It is not global, and only valid per servlet. It is used to pass initialisation parameters valid for only a specific servlet. |
Shared across all Servlets. |
Limited to a single servlet. |
Typically only one ServletContext per web application. |
Only one ServletConfig per servlet. |
It is used for global level parameters such as database config or global settings which many servlets want to share. |
Used for servlet level parameters, which has to be used on that specific servlet.. |
81. What is a thread lifecycle in Java?
There are 5 states in a thread lifecycle, let’s understand each one of them:
- New: The thread lies in the new state after it has been created. It remains in the new state until you start the execution process.
- Running: At runnable state, the thread is ready to run at any point in time or it might have started running already.
- Running: The scheduler picks up the thread and changes its state to running, the CPU starts executing the thread in the running state.
- Waiting: While being ready to execute, another thread might be running in the system. So, the thread goes to the waiting state.
- Dead: Once the execution of the thread is finished, its state is changed to the dead state, which means it’s not considered active anymore.
82. What are the different types of Exceptions in Java.
There are two types of Exceptions in Java Programming:
- Built-in Exception: These exceptions are the exceptions available in the standard package of java lang. They are used for certain errors with specific Exceptions as mentioned below:
- AriththmeticException: thrown for the errors related to the arithmetic operations.
- IOException: thrown for failed input-output operations.
- FileNotFourndException: raised when the file is not accessible or does not exist.
- ClassNotFoundException: exception is raised when the compiler is unable to find the class definition.
- InterruptedEException: raised when a thread is interrupted.
- NoSuchFieldException: raised when a class or method doesn’t have a variable specified.
- NullPointerException: thrown while referring to the variable or values of a null object.
- RuntimeException: raised for the errors occurring during the runtime. For example, performing invalid type conversion.
- IndexOutOfBoundsException: raised when the index of the collection like an array, string, or vector is out of the range or invalid.
- User-defined Exceptions: User-defined exceptions are the exceptions thrown by the user with custom messages. These are used for cases where the in-built Exception might not be able to define the error.
83. Can we write multiple try-catch block in java? If yes, how?
In Java, we can include multiple catch statements for a single try block. This is done, to handle different exceptions separately for a single try block.
Below is the program for multiple catch statements:
The output of the following program in different cases will be:
Case 1: Dividing an integer by zero
Enter the elements for division:
30
0
Arithmetic Exception occurred
End of the program
Case 2: Execution successful
Enter the elements for division:
15
3
Value of z: 5
End of the program
Case 3: Value entered is invalid
Enter the elements for division:
23
t
Any Exception in the try block
End of the program
84. What is the difference between throw and throws keyword?
Following are the key differences between the throw and throws keyword:
throw |
throws |
The throw keyword is used to explicitly throw an exception inside a program or a block of code. |
Throws keyword is used to declare an exception in the method signature that might occur during the compilation or execution of a program. |
Can throw only unchecked exceptions. |
Can be used for both checked and unchecked exceptions. |
throw keyword followed by an instance variable. |
throws keyword followed by the exception class names. |
throw keyword is used to throw only a single exception. |
throws can be used to throw multiple exceptions. |
85. Explain HTTP Tunneling?
HTTP tunneling refers to encapsulating of non HTTP data into HTTP/HTTPS. This is generally done to bypass firewalls, network restrictions or proxies where only HTTP or HTTPS traffic is allowed.
It works in a client-server setup:
- The client encapsulates the non-HTTP data into HTTP/HTTPS data and sends it through the firewall to the server.
- The server decrypts the encapsulated data, and forwards it to the destination. Once the response is received from the destination, the server again packs the data into HTTP/HTTPS and sends it to the client.
86. Explain the use of collections in Java.
A collection is a framework that provides a set of classes and interfaces, which are used to manage, store and manipulate data for a group of objects in Java.
Java Collections can be used for performing a variety of operations such as:
- Search
- Sort
- Manipulate
- Delete
Java Coding Interview Questions
87. How to write the string reversal program in Java without using the in-built function?
There are two ways we can reverse a string in java without using the in-built function:
- Using a loop by printing string backward, one character each time.
- Using a recursive function and printing the string backward.
In the following program, you can see both ways to print the string in reverse order:
88. What is a StringJoiner in java? Explain with a sample code.
StringJoiner is a class in Java that is used to join multiple strings together, optionally adding a delimiter between them. Here’s an example code that demonstrates the usage of StringJoiner: