Python is among the most popular programming languages today. Major organizations in the world build programs and applications using this object-oriented language. Here, you will come across some of the most frequently asked questions in Python coding interviews in various fields. Our Python developer interview questions for experienced and freshers will help you in your interview preparation. Let us take a look at some of the most popular and significant Python programming interview questions and answers:
The Python Interview Questions and Answers blog is classified into the following categories:
Python is the most used programming language in the world. Here are a few features of python that makes python different from other programming language:
Both Lists and Tuples are used to store collections of items, but they differ in meaningful ways.
The scope is the area in a program where a variable or a function is accessible. Simply put, it tells where to use or interact with a particular variable or function.
*args and **kwargs are passed as parameters in functions that help handle various arguments.
When an argument is passed to a function in Python, the argument is pointing to an object.
For example, When we call the function, the object’s reference is passed, and not the actual object. However, this behavior differs based on the mutability of the object.
If the object is mutable for example: a list, the reference to the actual object is passed. Hence, if the function modifies the object, the original object also gets modified.
As you can see from the example, the values of the object outside the function and inside the function change.
However, if the object is immutable, for example, an integer, if a change is made to the referenced object, the original object remains unaltered. However, a copy of the object is made inside the function and change happens to it. But the original object, that is outside the function the object’s value remains unaltered.
As you can see, the value of the object inside the function is different; however, the value of the object outside the function remains unaltered.
Lambda functions are also referred to as a “single-line function.” Here are a few characteristics of a lambda function:
Exceptional handling is a feature that helps developers handle errors and exceptions efficiently. It consists of multiple blocks, as stated below:
Point of Difference |
Sorted |
Sort |
Definition |
Returns a new list |
Modifies the list in place |
Scope |
Works on any iterable |
Works only on lists |
Syntax |
sorted(iterable, key=None, reverse=False) |
list.sort(key=None, reverse=False) |
Here is an example differentiating sorted() and sort():
18. What is compile time and runtime error? Do they exist in Python?
Compile time error and runtime error are the two errors that happen in Python at different stages of execution of the program.
Point of Difference |
Compile Time Error |
Runtime Error |
Definition |
Mistakes that are caught before the program runs. |
These are the errors that happen while your code is running after the syntax is checked and the program has started to execute. |
Type of error |
The errors identified at compile time are usually related to syntax |
The errors identified at runtime usually occur because something unexpected happened during the execution of the program that the Python interpreter wasn’t prepared for. |
Real-life Analogy |
You’re typing up an email and the spell checker underlines mistakes for you as you type |
You’re driving on a smooth road and suddenly hit a bump or a pothole |
Example |
x = 0
if x > 10 # Missing colon
print(“x is greater than 10”) |
number = 5
text = “hello”
result = number + text
>> TypeError: unsupported operand type(s) for +: ‘int’ and ‘str’ |
Yes, both types of errors exist in Python, but instead of using the term “compile-time error”, syntax errors are used more commonly in Python because these errors are caught when the interpreter is going through the code line by line and encounters a piece of code that doesn’t follow the syntax. Everything else is handled as runtime errors in Python. It includes errors like type errors, index errors, or exceptions that are only detected once the code is running.
Ride the Trend: Master Python and Stay Ahead in Tech!
Python Certification Training
19. What are generators and decorators?
Generators and Decorators are two methods used in Python to modify functions in a way that the code becomes more readable and efficient. However, they are used for completely different purposes.
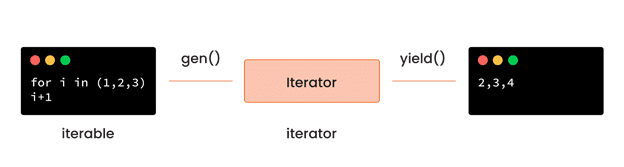
- Generators are functions that return an iterator. Using this iterator, you can iterate over the values one at a time.
- They use the yield method instead of the return method usually used in functions
- Useful when dealing with large datasets or infinite sequences
- Generator functions remember their state between each yield.
Here is an example representing use of generators:
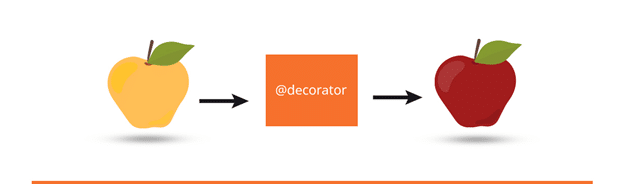
- Decorators are functions that modify an existing function or class.
- They extend the behavior or properties of the existing functions or classes without changing the code.
- Decorator functions take a function as an argument, add some behavior to it, and return the new function with modified or extended behavior.
- Useful in cases like logging, authentication, or timing how long a function takes to run
Here is an example of a decorator:
20. What is the difference between abstraction and encapsulation?
Abstraction and encapsulation are both a part of the 4 pillars of object-oriented programming, but they serve different purposes.
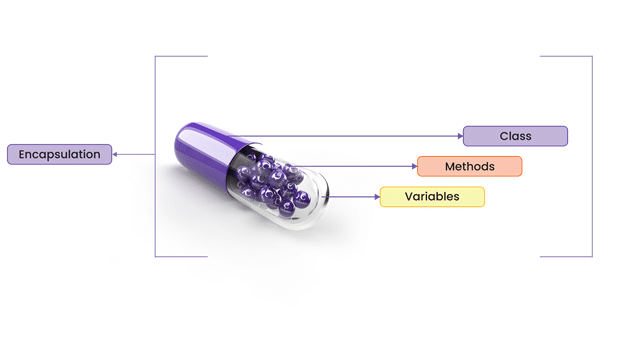
Point of Difference |
Abstraction |
Encapsulation |
Definition |
Hides the complexity of the system by showing only the essential details to the users. |
Restricts access to certain parts of an object to protect its details. |
Functionality |
Focuses on what an object does rather than how it does it |
Hides the internal details of an object by bundling the data or the attributes and the methods into a single unit, or class and making the attributes private. |
Application |
Users are exposed to the fact that pressing the brake pedal will stop the car rather than how the brake pedal stops the car. |
Similarly, all the internal mechanisms of the car are protected by making attributes like speed private so that they cannot be accessed publicly. |
Here is an example representing encapsulation:
Here is an example representing abstraction:
21. What is method overriding? How is it different from method overloading?
Method overriding and method overloading are two ways to achieve Polymorphism in object-oriented programming, but they work in different ways.
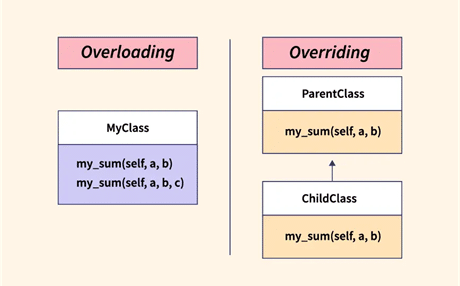
Method Overriding |
Method Overloading |
When a subclass provides a different implementation of a method that is already defined in its parent class. In other words, the child class overrides the behavior of the parent class’s method. |
Allows you to define several methods, each designed to handle a different number of inputs. |
Here is an example representing method overloading:
Here is an example representing method overriding:
22. How does inheritance work in Python?
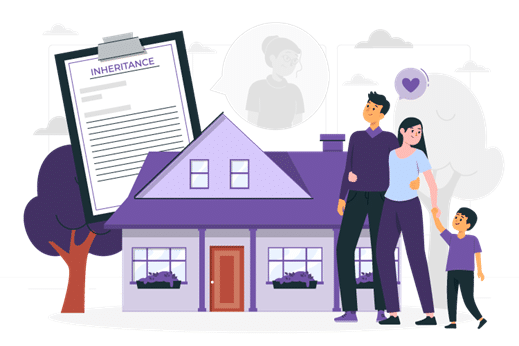
Inheritance is one of the 4 pillars of Object Oriented Programming. Inheritance allows one class to inherit aspects like attributes and methods from another class.
- The class that inherits is called the child class or sub-class.
- The class from which the attributes are inherited is called the parent class or superclass.
- On performing inheritance, the child class gets access to all the attributes and methods of the parent class.
- The child class can override, extend, or modify the methods of the parent class, promoting code reuse.
Here is an example of inheritance:
23. What is the significance of self in Python classes?
In Python, the self keyword acts as a bridge that connects an instance of a class with its methods and attributes.
- Whenever you create an object from a class you need a way to refer to that specific object inside the class’s methods.
- Real-life scenario: Employees have their names written on their badges. The self keyword is like the employee’s badge.
- Self refers to the object that calls the method, mapping the data with each object to specify the object’s data.
Example:
24. What are class methods and static methods? How are they different from instance methods?
Class methods, Static methods, and Instance methods are three different methods that follow different methods to interact with the class and its instances.
Class Methods
-
- Class Methods deal with the class itself
- They can modify the class-level attributes but not the objects or their instances.
- Class methods take “cls” as their first parameter rather than “self”, which refers to the class itself.
- Class methods are defined using the decorator @classmethod. This tells Python that the method works with the class rather than an instance
Static Methods:
-
- Static methods do not depend on any instance or class-specific data
- They are normal functions that are included inside the class for better code readability.
- Static methods are defined using the @staticmethod decorator
- They do not require any keyword like self or cls as their first parameter.
- Useful for implementing logic for a class but requires no access to the class or instance data.
Instance Methods
-
- Instance methods take self keyword as their first parameter
- They are used to access instance-specific data (attributes)
- They work with the individual object’s data
25. Explain the differences between a set and a dictionary.
Set and Dictionary are both built-in data structures for storing and managing data.
Point of Difference |
Set |
Dictionary |
Structure |
Elements are unordered but unique |
Stores data in the form of key-value pairs |
Purpose |
When you need a collection of unique items and don’t care about the order.
E.g., collecting unique words from a text. |
When you need to map one item to another.
E.g., storing people’s names (keys) against their phone numbers (values). |
Accessing elements |
You cannot access a particular element through indexing due to its unordered nature. |
Values can be accessed through the keys associated with the respective values. |
Here is an example implementing sets:
Here is an example implementing dictionary:
26. You are given a singly linked list that stores integer values in ascending order. Your task is to determine the time complexity for performing an insertion operation on numeric value 6 in the given LinkedList.
head -> 2 -> 5 -> 8 -> 12 -> None
The two main steps involved in an insertion operation in a singly LinkedList are Traversing and Inserting
- Traversing: Firstly, you need to find the right place to insert the element, which is between 5 and 8 in our case. Starting from the “head” node, you will traverse node by node till you find the location. The time complexity for this operation will depend on the number of nodes O(n).
- Comparing: As you traverse through the nodes, you have to compare each node with the element you want to insert. If the element is smaller than the current node element, you will insert the new node else move to the next node. This process is repeated until you reach the end of the list and it will have a complexity of O(1).
- Inserting: Once you have located the position where you want to insert, you will adjust the pointers in a way such that your previous node which is the node containing 5, will have the next pointer pointing to the new node containing 6.You then need to point the next pointer of your new node to the node containing 8. This operation also requires a constant time, i.e. O(1)
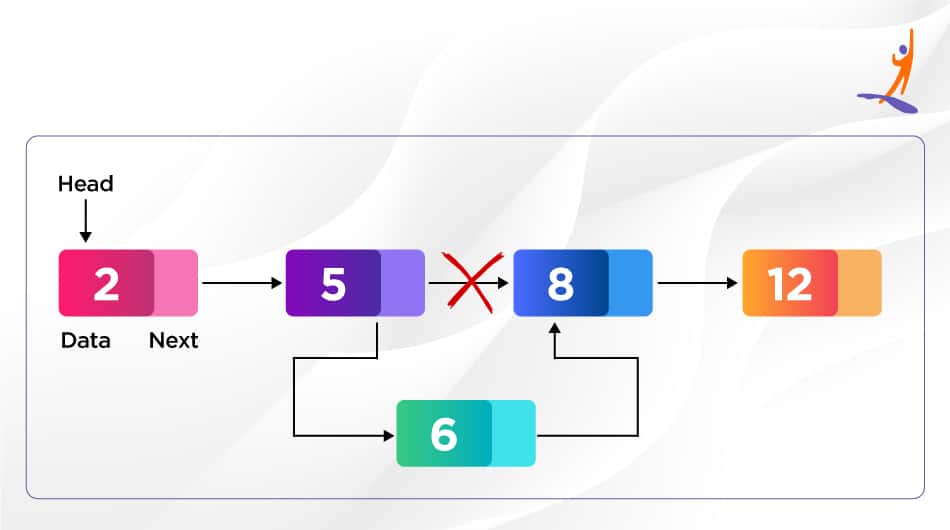
So the overall time complexity of inserting an element in the LinkedList at a random position is O(n).
27. How do you find the middle element of a linked list in one pass?
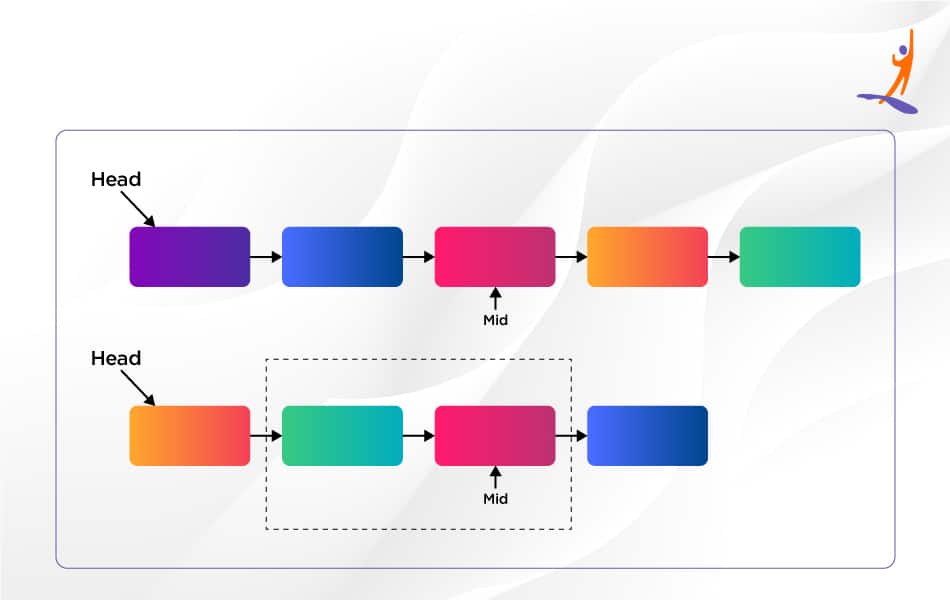
Here the main catch in the question is “one pass”. What does it mean? It means you get to traverse the list only once and there’s no coming back to a node once you’ve traversed it. But to calculate the middle element you will require the length of the entire LinkedList, right?
So this is where the Two-Pointer Technique also known as the Slow and Fast Pointer method.
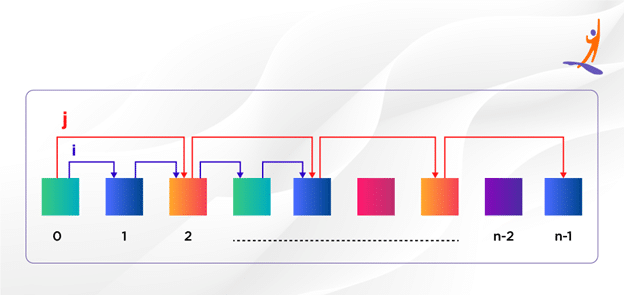
You will have 2 pointers one moving twice as fast as the other. In other words, the fast pointer will skip a node and jump to second node when the slow pointer The main logic behind having the two pointers is that at the time the fast pointer reaches the end of the list, the slow pointer will be at the middle node.
Now the question here is that how do we know that the fast pointer has reached the end of the list?
This is implemented through the logic “while fast and fast.next” which basically means that we will iterate through the list only if our fast pointer is not null.
So the moment the fast pointer reaches the null node, the condition remains unsatisfied and the loop is terminated.
Edge Case:
Even number of nodes: If the list has an even number of nodes, you will have to explicitly decide what “middle” means for your problem because you will have two middle elements in the list. You can return either of the two elements depending on the problem and sample outputs provided.
28. You have a string, and you want to find the length of the longest substring of that string where no characters repeat. For example, in the string
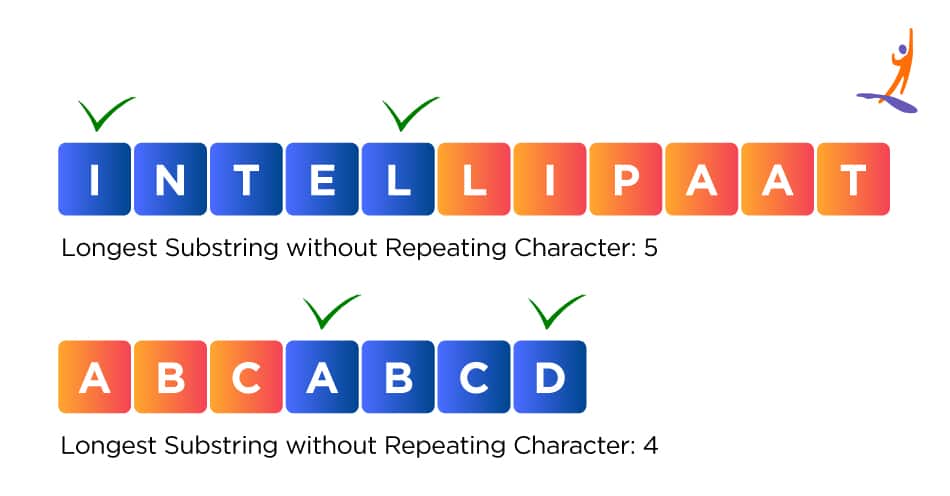
For implementation-based questions like this one, the interviewer is not looking if you can write a code for the problem but rather if you can come up with an optimized solution and get the logic right for it.
Your first instinct would be to blurt out the answer saying “Generate all the possible substrings and then look for the substring having all unique characters and also the maximum length.”
This approach is not wrong but as we mentioned, this is not what the interviewer is looking for.
The right answer would be an optimized logic like the sliding window, where you would explain the main concepts like:
- Two Pointers: You would use two pointers, left and right, which represent the start and end of your current window or substring. Your right pointer will be traversing the characters of the string one at a time, expanding your window or your substring.
- Track Seen Characters: To check for duplicates, you will need a hashmap or a dictionary to store all the characters in the current window along with their index values.
Every time your right pointer moves to the next index, you have to check whether the character is already present in your dictionary.
If it is present, it is a duplicate character, and now instead of incrementing the right pointer, you will increment the left pointer by 1 plus the index value of the duplicate character where it occurred last.
If it is not a duplicate character, you will add it to the dictionary and increment your right pointer.
At this point, our dictionary or hashmap will look something like this:
a -> 0 3
b -> 1 4
c -> 2 5
d ->
Looking at this map, you should be able to figure out that lastly, d will get mapped with index 6, and since it is not a repeating character, our maximum length will get updated to 4, and hence we get our final answer.
- Check for Maximum Length: Each time you adjust the window containing all unique elements, you need to check if the length is greater than your previous window length. The formula for it is max(max_len, right – left + 1).
29. You're given a set of items, each with a weight and value. You are required to figure out how to pack the most valuable items into a knapsack without exceeding its weight limit, where each item can either be taken or left behind.
This problem is also known as 0/1 Knapsack problem. You are given a Knapsack with a certain weight limit or capacity. Keeping this capacity in mind, you need to fill the items in your knapsack in such a way that you maximize the total value of the items.
We will not look at the Brute Force approach but rather directly dive into the optimized solution which will be achieved using Dynamic Programming.
We will understand this with the help of an example.
We are given 4 items, where List A gives the price of the items and List B gives the weights of the items respectively and variable C gives the capacity of the bag or the knapsack.
Price (A) = {1, 2, 5, 6}
Weights (B) = {2, 3, 4, 5}
Bag capacity (C) = 8
|
|
0 |
1 |
2 |
3 |
4 |
5 |
6 |
7 |
8 |
Profit |
Weight |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
1 |
2 |
0 |
0 |
1 |
1 |
1 |
1 |
1 |
1 |
1 |
2 |
3 |
0 |
0 |
1 |
2 |
2 |
3 |
3 |
3 |
3 |
5 |
4 |
0 |
0 |
1 |
2 |
5 |
5 |
6 |
7 |
7 |
6 |
5 |
0 |
0 |
1 |
2 |
5 |
6 |
6 |
7 |
8 |
We will create a table where rows (i) represent each item, and columns (j) represent different possible weight capacities up to the maximum weight. Let’s call this table V. Here, V[i][j] will represent the maximum value that can be achieved with the first i items and a weight limit of j.
Initialize the Table:
- If no items are chosen (i.e., i = 0), the maximum value for any weight w is 0 because there are no items to include.
- If the weight limit is 0, the maximum value is also 0 because the knapsack can’t hold anything.
Fill in the Table:
- If the item’s weight is greater than j, we can’t include it, so V[i][j] = V[i-1][j]
- If the item’s weight is less than or equal to w, you have two options:
- Exclude the item: V[i][j] = dp[i-1][j]
- Include the item: dp[i][j] = value[i] + dp[i-1][j – weight[i]]
- You need to find the maximum value out of the two options because the maximum one is going to be more profitable.
V[i,j] = max{V[i-1,j], V[i-1, j-wi] + Pi}
Find the result: After filling out the table, the answer will be in V[n][W], which is the last cell of the table. Here, n is the number of items and W is the knapsack’s weight limit. This cell gives the maximum profit that can be achieved using the items given and keeping in mind the knapsack’s weight limit.
Get 100% Hike!
Master Most in Demand Skills Now!
30. You are given a series of asteroids moving in a line; when they collide, they either destroy each other or continue moving. You have to determine the final state of all the asteroids after all collisions.
You will be given a list of integers representing the magnitudes of the asteroids. An integer having positive magnitude is considered to be moving in the right direction whereas a negative magnitude asteroid moves in the left direction.
We will use a stack data structure, to keep track of asteroids that haven’t yet collided and in the end, we will just return the stack representing the final state of the asteroids left or the asteroids that did not get destroyed after all the collisions.
- Iterate Through Each Asteroid:
- If an asteroid is moving right (positive), add it to the stack since there’s no chance of a collision
- If an asteroid is moving left (negative), check for a collision with the asteroid at the top of the stack
- Handle Collisions:
- If the stack is not empty and the top of the stack element is moving right:
- If the left-moving asteroid has a greater magnitude than the top of the stack element, the top of the stack element will get popped from the stack since it has been destroyed. The collision will continue with the next asteroids.
- If the right-moving asteroid has a greater magnitude, it will destroy the left-moving asteroid. We simply move to the next asteroid.
- If they’re equal in magnitude, both the asteroids get destroyed, i.e.: we pop the top of the stack element and also move on to the next asteroid.
- Continue this process until there are no more asteroid collisions.
- Result:
- After iterating through all the asteroids, all the asteroids that survived the collisions are in the stack. These are the final asteroids remaining after all the collisions.
E.g: You are given a list of asteroids: [ 5, 10, -5, -15]
- We will iterate through the list of asteroids and check for collisions one by one. Since our stack is empty, we add the first asteroid we come across in the stack, which is 5. Then we move to the next asteroid which is 10. Since 5 and 10 are moving in the same direction, there will not be any collision and hence 10 will also be pushed in the stack.
- Then we go ahead and compare the next asteroid which is -5 and the top of the stack element -> 10. Since they are moving in opposite direction and 10 is greater in magnitude than -5, -5 asteroid will get destroyed. So we simple move to the next asteroid skipping the current asteroid (-5)
- Then we go ahead and compare the next asteroid which is -15 with the top of the stack element (10). Since -15 is greater in magnitude than 10, the top of the stack element, 10, will get destroyed which means we will pop the element.
- Then we compare our new top of the stack element, which is 5 with -15. Since -15 again has a greater magnitude than 5, we will pop the element since it will get destroyed.
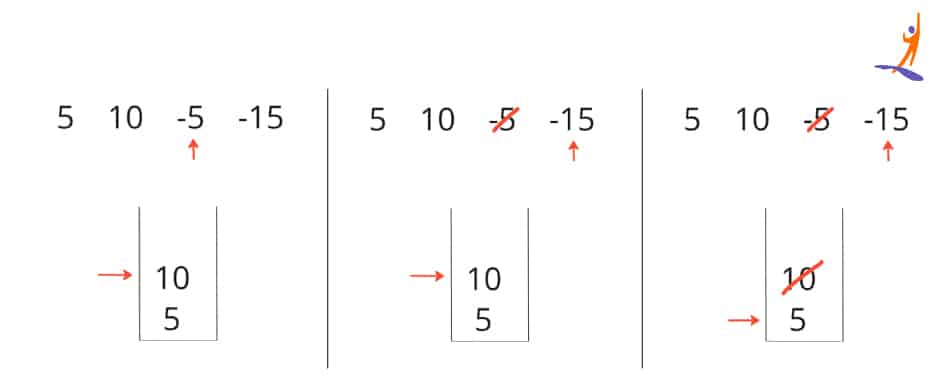
Finally, we will be left with -15 element in the stack. We will return the stack as our final output which shows the final state of all the asteroids after the collisions. In our case, we are only left with -15 which means, one asteroid having magnitude 15 moving in the left direction.
CTA
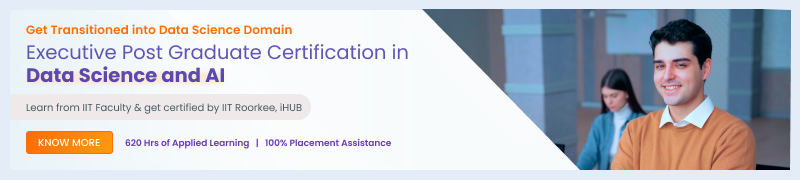
31. What is Python, and how does its dynamic typing work?
Python is a high-level, general-purpose programming language developed by Guido van Rossum in 1991. It utilizes English keywords extensively and has a simpler syntax as compared to multiple other programming languages.
It’s a dynamically typed language with capabilities of garbage collection for better memory performance. It supports multiple programming paradigms, including structured, object-oriented, and functional programming. Dynamic typing in Python allows you to determine the type of a variable at runtime rather than during code compilation.
Example:
Here, x can hold different types of data (first an integer, then a string) during its lifecycle. Python internally tracks the type of x and adjusts its behavior based on the current value.
32. What are loops in Python? How do you write a nested for-loop program?
The loop is a sequence of instructions that gets executed repeatedly until and unless an exit condition is reached.
There are two types of loops in Python:
- For Loop: This Loop iterates over a sequence like lists, strings, tuples or any other iterable object and is used when the number of iterations is known in advance.
- While Loop: A while loop continues executing as long as the specified condition is true. It’s useful when the number of iterations is not known in advance.
- Nested For-Loop in Python: A nested for-loop is a loop inside another loop. It becomes useful when you need to iterate over multiple dimensions, like rows and columns of a matrix.
33. What are collections? What is the significance of collections in Python?
Collections refer to data structures or containers capable of holding collective data in a single variable. These data structures are alternatives to built-in types such as lists, dictionaries, sets, and tuples
Let’s take an example to understand it. Suppose you have lots of books and are trying to build a library. Each book is different; some are about life lessons, some are about stories, and some are about magic. In Python, collections are like those collections of books. They help you organize lots of things (like numbers, words, or other data) in an organized way, just like how you organize your books in your library.
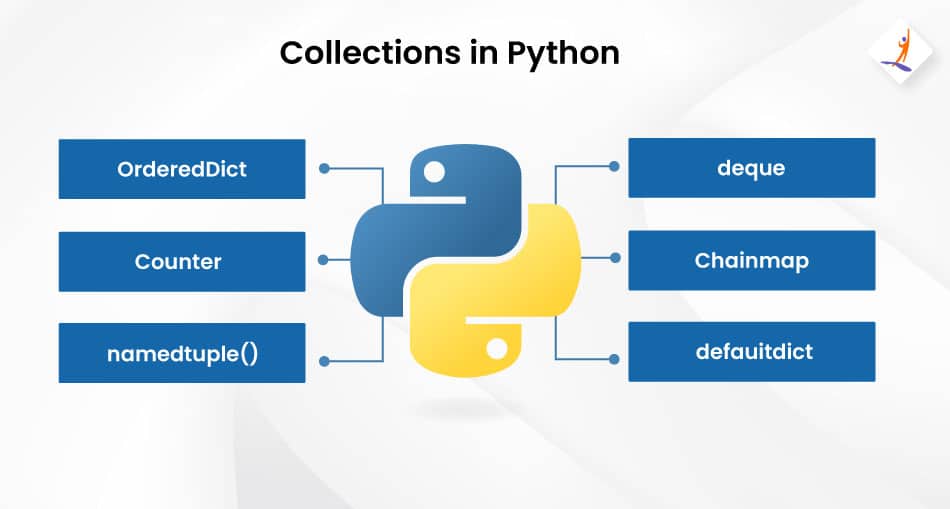
Collection Module implements specialized container data types, providing alternatives to Python’s general-purpose built-in containers.
- namedtuple: A tuple subclass that allows accessing elements by name and index. It provides a more readable and self-documenting structure.
- deque: A deque or double-ended queue allows efficiently adding and removing elements from both ends, which is useful for implementing queues and stacks.
- Counter: A dictionary subclass for counting hashable objects, which is used to count occurrences of elements in a collection like a list or string.
- defaultdict: It is a dictionary subclass that provides a default value for a nonexistent key. This is useful when you want to avoid KeyError and automatically initialize missing keys.
- OrderedDict: A dictionary subclass that maintains the order of keys as they are added. In Python 3.7+, regular dictionaries maintain insertion order by default, but OrderedDict ensures this behavior in earlier versions and provides extra methods.
- ChainMap: A class that groups multiple dictionaries or mappings into a single view
34. What is the difference between %, /, //?
In Python, %, /, and // are arithmetic operators with distinct functions:
- The ‘ % ’ is the modulus operator, which returns the remainder of a division. For instance, 5 % 2 would return 1.
- The ‘ / ’ is the division operator that performs floating-point division and returns a float. For example, 5 / 2 would return 2.5.
- The ‘ // ’ is the floor division operator that performs division but rounds down the result to the nearest whole number. So 5 // 2 would return 2.
35. What is the method to write comments in Python?
Python comments are statements used by the programmer to increase the readability of the code. With the help of the #, you can define a single comment. Another way of commenting is to use the docstrings (strings enclosed within triple quotes).
36. Do we need to declare variables with respective data types in Python?
No, Python is a dynamically typed language, and Interpreter automatically identifies the data type of a variable based on the type of value assigned.
37. What distinguishes lists from tuples?
Here are the major differences between List and Tuple:
Lists |
Tuple |
Lists are mutable, i.e., they can be edited |
Tuples possess immutability, denoting their incapability of being modified like lists. |
Lists are usually slower than tuples |
Tuples are faster than lists |
Lists consume a lot of memory |
Tuples consume less memory comparatively |
Lists have a higher likelihood of experiencing unexpected changes, making them less reliable in terms of errors |
Tuples offer increased reliability due to their resistance to unexpected modifications |
38. Is this statement true: “Python is a case-sensitive language”?
Yes, Python is a case-sensitive language. For example – “Function” and “function” are distinct entities.
39. What is the method for generating random numbers in Python?
Random Module is used to generate a random number in python
40. What does len() do?
The len() function is a built-in method that is used to get the length of sequences such as lists, strings, and arrays.
41. What is file handling in Python? What are the various file-handling operations in Python?
File handling is a technique that handles file operations such as the process of opening, reading, writing, and manipulating files on the file system.
Python File Handling Operations can be categorized into the following categories:
- ‘r’: Read (default mode).
- ‘w’: Write (creates a new file or truncates an existing file).
- ‘a’: Append (adds content to the end of an existing file).
- ‘b’: Binary mode (used for binary files).
42. What is PEP 8?
PEP in Python stands for Python Enhancement Proposal. It comprises a collection of guidelines that outline the optimal approach for crafting and structuring Python code to improve the readability and clarity of the code.
43. What is PYTHONPATH?
PYTHONPATH is an environment variable in Python that specifies a list of directories where the Python interpreter looks for modules and packages to import. By default, Python searches for modules in the current directory and the standard library directories, but you can extend this search path using the PYTHONPATH variable.
44. What are Python namespaces?
It ensures the names assigned to objects within a program are unique and can be used without conflict. In Python, namespaces has a unique name for each object in Python.
Here is a list of namespaces in python:
- The Local Namespace is specific to a function and contains the names defined within that function. It is created temporarily when the function is called and is cleared once the function finishes executing.
- The Global Namespace includes names from imported modules or packages that are used in the current project. It is created when the package is imported into the script and remains accessible throughout the script’s execution.
- The Built-in Namespace comprises the built-in functions provided by Python’s core, as well as specific names dedicated to various types of exceptions.
45. Explain the difference between pickling and unpickling.
Here’s a comparison of pickling and unpickling in table format:
Aspect |
Pickling |
Unpickling |
Definition |
The process of converting a Python object into a byte stream. |
The reverse process of converting a byte stream back into the original Python object. |
Purpose |
To save the state of an object for storage or transmission. |
To retrieve and reconstruct the original object from its serialized format. |
Usage |
– pickle.dump(obj, file) writes an object to a file.
– pickle.dumps(obj) returns a byte stream. |
– pickle.load(file) reads an object from a file.
– pickle.loads(byte_stream) reconstructs an object from a byte stream. |
Example Function |
pickle.dump(data, file) |
loaded_data = pickle.load(file) |
46. What do you understand about iterators in Python?
Iterators are objects that allow us to traverse through a collection (such as lists, tuples, dictionaries, or sets). They use the __iter__() and __next__() methods to retrieve the next element until there are no more. Iterators are commonly used in for loops and can be created for custom objects. They promote efficient memory usage and enable lazy evaluation of elements.
47. What is Experimental Just-in-Time (JIT) Compilation in Python
Experimental Just-in-Time (JIT) Compilation in support in Python 3.14 with CPython as the main interpreter. which is known as an upcoming performance enhancement feature introduced. The goal of JIT is to improve the execution speed of Python code by compiling parts of it during runtime instead of interpreting it line-by-line, as is the case traditionally.
48. Can we use else with For in Python?
Yes, the else clause is an integral part of the for-loop. It is executed when the loop completes normally and no condition is TRUE.
Python Interview Questions For Experienced
49. What is the purpose of using super() in Python classes?
The super() function in Python is used to call methods from a parent within a subclass.
50. Explain the use of yield in Python with an example.
The yield keyword is used to create a generator function, which is used to return a list of values from a function.
51. What is multi-threading in Python?
Multithreading is a technique where processors execute multiple threads concurrently within a single process. A thread is the smallest unit of a CPU’s execution that can run independently. By using multiple threads, a program can perform several tasks simultaneously, which can improve performance, particularly for I/O-bound tasks.
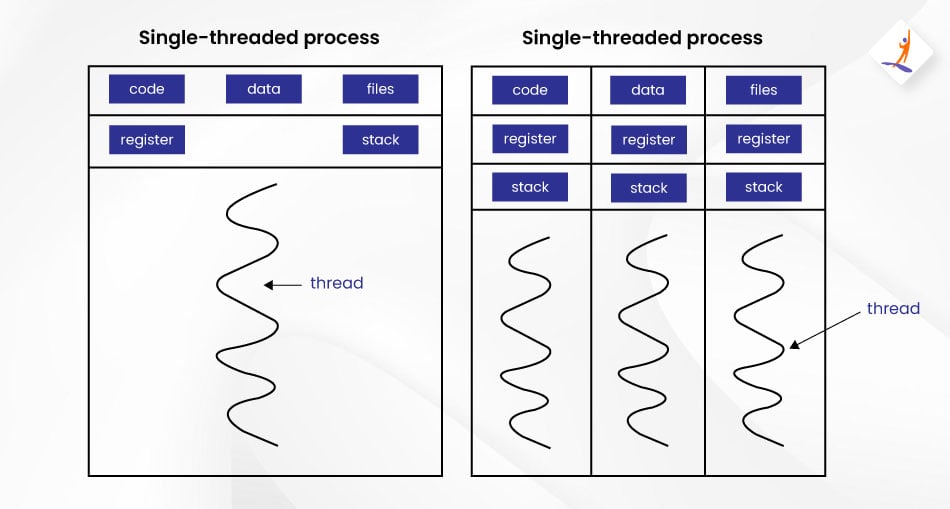
52. What are Access Specifiers in Python?
Access specifiers (also known as access modifiers) are used to control the visibility or accessibility of class attributes and methods.
- Public members: Can be accessed from anywhere.
- Protected members: Signaled by a single underscore _, indicating they should only be accessed within the class or subclasses.
- Private members: indicated by a double underscore __, which makes the member less accessible outside the class.
53. Does Python support multiple inheritance?
Yes, Python supports multiple inheritance, which means a class can inherit from more than one parent class. In multiple inheritance, a class can have multiple base classes, and it inherits attributes and methods from all of them.
54. Does Python support Switch Case?
No, Python does not have a built-in switch statement like some other languages (such as C or Java). Instead, Python developers typically use Match and Case, if-elif-else chains, or dictionary mappings to achieve similar functionality.
55. What is Walrus Operator?
The Walrus Operator (:=) is a new feature introduced in Python 3.8. It allows you to assign a value to a variable and return the value within a single expression. This is known as assignment expression
Python OOPS Interview Questions
56. What are the four pillars of OOP? Explain each
Here are the main four pillars of OOPs:
- Inheritance allows us to inherit the behaviors or properties from the parent class to the child class.
- Polymorphism means “many forms” and in programming functions with the same name that can be executed on many objects or classes for different behavior.
- Encapsulation is the practice of bundling the data (attributes) and methods (functions) that operate on the data into a single unit (class) and restricting direct access to some of the object’s components.
- Abstraction is the process of hiding implementation details and showing only essential features of an object.
57. What is a class and an object in Python
A class is a blueprint for creating objects. It contains member functions, attributes, etc., that get instantiated when the object is called.
On the other hand, an object is nothing but an instance of a class, possessing state, identity, and functionality, and is used to call class members.
Let’s take a look at a simple example:
58. What are constructors?
Constructors are called when an object is created for a class. Constructors are used to instantiate the objects and assign values to them.
59. What is abstraction?
One of the pillars of object-oriented programming is abstraction. Abstraction is a very simple process where only the necessary details are shown, and the background computations or processes stay hidden.
To simplify, let’s try to understand abstraction with an example:
Let’s say you visit a motor showroom to buy your new car. The dealer will take you for a quick ride to show you the features of the car.
The noticeable thing here is that you will be shown the entire car, but you will not be able to figure out how the actual combustion and other necessary details to move the car are working. This is exactly how abstraction works: only the necessary details are shown, and internal functionality is hidden from the users.
Python Coding Interview Questions
60. Write a program in Python to find the largest and second-largest element in a list using Python.
Here the problem statement says that we have to find out the largest and second-largest element from a list containing.
61. Write a program in Python to produce a Star triangle
The below code produces a star triangle-
62. Write a program to produce the Fibonacci series in Python
The Fibonacci series refers to a series where an element is the sum of two elements before it.
63. Write a program in Python to check if a number is prime
The below code is used to check if a number is prime or not
64. Write a program to check even odd numbers using shorthand if-else statements.
Let’s first understand even and odd numbers. When can a number be even? A number is even when divided by two and returns a remainder zero. Now we know that the remainder can be determined with the help of the modulus function (%), which returns the remainder of the division. Now, let’s go ahead and write the program.
65. Write a Python program to count the total number of lines in a text file.
This Python program defines a function to count lines in a text file. It opens the file, uses a generator expression to count lines efficiently, and handles errors for missing files. The function returns the total line count, and an example usage demonstrates how to call it with a file path.
66. Write a program in Python to execute the Bubble sort algorithm.
67. What is monkey patching in Python?
Monkey patching is the term used to denote modifications that are done to a class or a module during runtime. This can only be done as Python supports changes in the behavior of the program while it is being executed.
The following is an example, denoting monkey patching in Python:
The above module (monk) is used to change the behavior of a function at runtime as shown below:
68. Write a Program to print the ASCII Value of a character in Python.
We can can print the ASCII value of a character in Python using the built-in ord() function.
69. How will you reverse a list in Python?
There are multiple ways to reverse a list. Here are a few of them listed:
- Using reverse() method
- Using slicing technique
- Using reversed() function
- Using insert() function
70. Write a program to Reverse a list using Enumerate in Python.
Reverse a list using the enumerate() function in Python by iterating over the original list and creating a new list with the elements in reverse order.
71. Write a Python program to print a list of primes in a given range.
In this program where we need to check if the number is a prime number or not, we are making use of a very simple approach. Firstly we need to understand what is a Prime Number. A whole number greater than 1 cannot be exactly divided by any whole number other than itself and 1.
72. Write a Python program to check whether the given input is an Armstrong number.
73. Write a program to find the greatest of the two numbers.
74. Write a Python program to check whether a given string is a palindrome without using an iterative method.
A palindrome is a word, phrase, or sequence that reads the same backward as forward, e.g., madam.
75. What is the easiest way to calculate percentiles when using Python?
The easiest and most efficient way to calculate percentiles in Python is to use NumPy arrays and their functions.
76. Write a Python program that removes duplicates from a list
Removing duplicates from a list can be done easily by converting the list into a set and then moving it back to a list. As it is a property of a set that can only contain unique.
77. Write a Python program that will reverse a string without using the slicing operation or reverse() function.
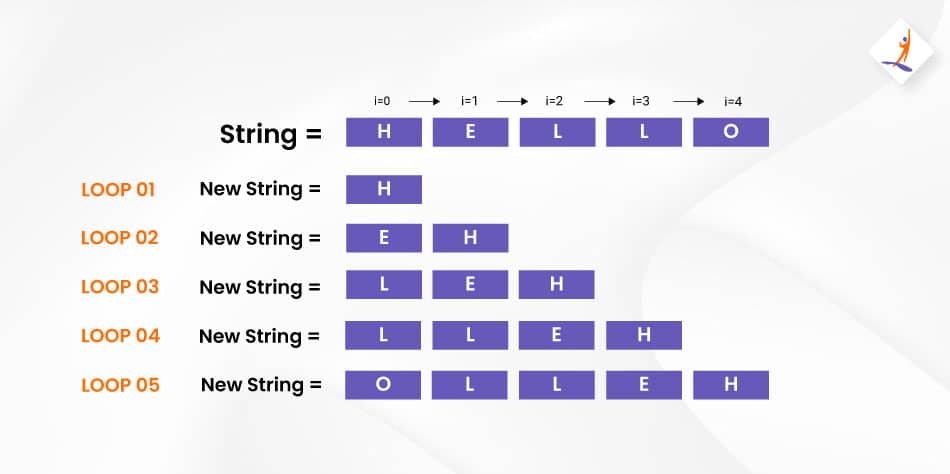
78. What is the difference between range & xrange?
range() and xrange() are used to iterate in a for loop for a fixed number of times. Functionality-wise, both these functions are the same. The difference comes when talking about Python version support for these functions and their return values.
range() Method |
xrange() Method |
In Python 3, xrange() is not supported; instead, the range() function is used to iterate in for loops |
The xrange() function is used in Python 2 to iterate in for loops |
It returns a list |
It returns a generator object as it doesn’t really generate a static list at the run time |
It takes more memory as it keeps the entire list of iterating numbers in memory |
It takes less memory as it keeps only one number at a time in memory |
79. Write a command to open the file c:\hello.txt for writing?
f= open(“hello.txt”, “wt”)
80. How will you remove duplicate elements from a list?
To remove duplicate elements from the list, we use the set() function.
Consider the below example:
81. How can files be deleted in Python?
You need to import the OS module and use os.remove() function for deleting a file in Python.
import os
os.remove("file_name.txt")
82. What would be the output if I run the following code block?
list1 = [2, 33, 222, 14, 25]
print(list1[-2])
A) 14
B) 33
C) 25
D) Error
The correct output will be 14, i.e. Option A, because here we have a list and negative indexing is used on it. Negative indexing starts from the end with -1. So if you count, then 14 is the second last element that will get printed.
83. Whenever Python exits, why isn’t all the memory de-allocated?
- Whenever Python exits, especially those Python modules which are having circular references to other objects or the objects that are referenced from the global namespaces are not always de-allocated or freed.
- It is not possible to de-allocate those portions of memory that are reserved by the C library.
- On exit, because of having its own efficient clean-up mechanism, Python would try to de-allocate every object.
84. How can the ternary operators be used in python?
Ternary operator is the operator that is used to show the conditional statements in Python. This consists of the boolean true or false values with a statement that has to be checked .
Syntax:
[on_true] if [expression] else [on_false]x, y = 10, 20 count = x if x < y else y
Explanation:
The above expression is evaluated like if x<y else y, in this case if x<y is true then the value is returned as count=x and if it is incorrect then count=y will be stored to result.
85. How to add values to a python array?
In Python, adding elements to an array can be easily done with the help of extend(), append(), and insert() functions.
Consider the following example:
86. How to remove values to a python array?
Elements can be removed from the python array using pop() or remove() methods.
pop(): This function will return the removed element .
remove(): It will not return the removed element.
Consider the below example :
87. How will you remove the last object from a list in Python?
Here, −1 represents the last element of the list. Hence, the pop() function removes the last object (obj) from the list.
88. How will you convert a string to all lowercase?
lower() function is used to convert a string to lowercase.
89. What is the difference between append() and extend() methods?
Both append() and extend() methods are methods used to add elements at the end of a list.
- append(element): Adds the given element at the end of the list that called this append() method
- extend(another-list): Adds the elements of another list at the end of the list that called this extend() method
90. How do you identify missing values and deal with missing values in Dataframe?
isnull() and isna() functions are used to identify the missing values in your data loaded into dataframe.
missing_count=data_frame1.isnull().sum()
There are two ways of handling the missing values :
Replace the missing values with 0
df[‘col_name’].fillna(0)
Replace the missing values with the mean value of that column
df[‘col_name’] = df[‘col_name’].fillna((df[‘col_name’].mean()))
91. What is regression?
Regression is termed as supervised machine learning algorithm technique which is used to find the correlation between variables and help to predict the dependent variable(y) based upon the independent variable (x). It is mainly used for prediction, time series modeling, forecasting, and determining the causal-effect relationship between variables.
Scikit library is used in Python to implement regression and all machine learning algorithms.
There are two different types of regression algorithms in machine learning:
Linear Regression: Used when the variables are continuous and numeric in nature.
Logistic Regression: Used when the variables are categorical in nature.
92. What are python module and libraries?
Python has a rich set of libraries. Libraries or modules refer to a set of predefined functions that are packed together in the form of packages. These are ready-to-use functions. You have to import the library and make a function call to use those functions.
93. What are the different types of inheritance in python?
Inheritance is a major pillar in the OOPs concept. There are five types of inheritance available in Python. All of them are listed below:
- Single Inheritance: Situation where a class inherits properties from one superclass.
- Multiple Inheritance: Situation where a class inherits properties from multiple superclass
- Multilevel Inheritance: Situation where a class inherits properties from a superclass and that superclass is inheriting properties from another superclass.
- Hirerical Inheritance: Situation where multiple classes are inheriting properties from a single superclass.
- Hybrid Inheritance: Situation where multiple different types of inheritance are used.
94. What is a dictionary in Python?
Dictionary is one of the collective data types available in Python. It has a few characteristics:
- It can store a set of heterogeneous elements (elements with different data types).
- It stores the elements in the form of a key-value pair.
- No order is maintained while storing the elements, i.e., indexing and slicing are not applicable.
- Keys can’t be collective datatypes except for strings.
Syntax:
Variable_name = {key1 : value1, key2 : value2, ………}
Example:
95. What are functions in Python
Functions are entities in Python that increase code resusability and decrease code base size. These are reusable blocks of code that can be invoked by just making a function call. A function consists of two parts:
Function Definition: It is where you define what the function will perform
Function Call: It is used to invoke the function
96. What are the common built-in data types in Python?
Here are the set of built in data types in python:
Universal Data type:
- Integer refered as int
- Float refered as float
- Boolean refered as bool
Collective Data Type:
- Lists
- String
- Tuple
- Dictionary
- Set
97. What is type conversion in Python?
Type conversion also known as type casting is the process of converting a variable from one data type to another. This is done by passing the variable as a parameter in the data type class function.
For Example:
98. What does [::-1] do?
It is a slicing notation that is used to reverse the sequence. Lets break it down
The syntax for slicing is [start: stop: step]. We know that if start and stop are not mentioned, then it takes the default value of start and stop. So the slicing notation is [0:n:-1] (n refers to the last index), which basically mean traverse the whole data but with step -1, i.e reverse order (negative indexing)
99. Is indentation required in Python
Yes, indentation is very important in Python. If indentation is not provided properly, then the program will throw an error stating “indentation error.”.
100. How can you randomize the items of a list in Python
To randomise the items in a list in Python, we use the shuffle() from the random module. Make sure to import it. Here is an example:
101. How to comment with multiple lines in Python?
To comment multiple lines in Python, every line should be prefixed with #. To do so select the lines that you want to comment and press CTRL + / from your keyword. This will comment on all the selected lines. To uncomment it, use the same shortcut.
102. What type of language is python? Programming or scripting
Python is a general-purpose programming language. It can be used for programming as well as scripting.
103. Differentiate between NumPy and SciPy?
NumPy and SciPy are two different libraries extensively used in python. Here are the differences between NumPy and SciPy:
NumPy |
SciPy |
The NumPy library mostly consists of manipulation functions that can help you with tasks such as creating data structures and manipulating the data in them. |
SciPy is built on the numPy extension, wherein it is used to solve scientific mathematics using a wide range of inbuilt functions. |
104. Explain the use of the 'with' statement and its syntax?
The with statement is used to open a file and close it as soon as it exits the block of code. Here is the syntax:
with open("filename", "mode") as file_var:
105. How will you read a random line in a file?
Here is the code to read a random line from a file:
import random
def read_random(fname):
lines = open(fname).read().splitlines()
return random.choice(lines)
print(read_random (‘hello.txt’))
106. Why would you use NumPy arrays instead of lists in Python?
Here are a few reasons to use NumPy arrays instead of list:
- NumPy arrays is highly readable due to its simple syntax.
- NumPy arrays consume less memory as compared to a list hence it is more efficient
- NumPy arrays are efficient so, it is light in processing
CTA
These Python Developer interview questions will help you land the following job roles:
- Python Developer
- Research Analyst
- Data Analyst
- Machine learning engineer
- Software Engineer
- Data Scientist
Our Python Courses Duration and Fees
Cohort starts on 14th Jan 2025
₹20,007
Cohort starts on 21st Jan 2025
₹20,007